In this blog, we will discuss designing contact us page using asp.net and c#. It's very common for web sites to contain a contact us page which allows the users of the site to contact either the administrator or the support group. A typical contact-us page is shown below.
contactus.aspx:
<div style="font-family: Arial">
<fieldset style="width: 350px">
<legend title="Contact us">Contact us</legend>
<table>
<tr>
<td>
<b>Name:</b>
</td>
<td>
<asp:TextBox
ID="txtName"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator1"
runat="server"
ControlToValidate="txtName"
ErrorMessage="Name is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td>
<b>Email:</b>
</td>
<td>
<asp:TextBox
ID="txtEmail"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
Display="Dynamic"
ForeColor="Red"
ID="RequiredFieldValidator2"
runat="server"
ControlToValidate="txtEmail"
ErrorMessage="Email is required"
Text="*">
</asp:RequiredFieldValidator>
<asp:RegularExpressionValidator
Display="Dynamic"
ForeColor="Red"
ID="RegularExpressionValidator1"
runat="server"
ErrorMessage="Invalid Email"
ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"
ControlToValidate="txtEmail"
Text="*">
</asp:RegularExpressionValidator>
</td>
</tr>
<tr>
<td>
<b>Subject:</b>
</td>
<td>
<asp:TextBox
ID="txtSubject"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator3"
runat="server"
ControlToValidate="txtSubject"
ErrorMessage="Subject is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="vertical-align: top">
<b>Comments:</b>
</td>
<td style="vertical-align: top">
<asp:TextBox
ID="txtComments"
Width="200px"
TextMode="MultiLine"
Rows="5"
runat="server">
</asp:TextBox>
</td>
<td style="vertical-align: top">
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator4"
runat="server"
ControlToValidate="txtComments"
ErrorMessage="Comments is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td colspan="3">
<asp:ValidationSummary
HeaderText="Please fix the following errors"
ForeColor="Red"
ID="ValidationSummary1"
runat="server" />
</td>
</tr>
<tr>
<td colspan="3">
<asp:Label
ID="lblMessage"
runat="server"
Font-Bold="true">
</asp:Label>
</td>
</tr>
<tr>
<td colspan="3">
<asp:Button
ID="Button1"
runat="server"
Text="Submit"
OnClick="Button1_Click" />
</td>
</tr>
</table>
</fieldset>
</div>
contactus.aspx.cs
public partial class contactus : System.Web.UI.Page
{
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (Page.IsValid)
{
MailMessage mailMessage = new MailMessage();
mailMessage.From = new MailAddress("YourGmailID@gmail.com");
mailMessage.To.Add("AdministratorID@YourCompany.com");
mailMessage.Subject = txtSubject.Text;
mailMessage.Body = "<b>Sender Name : </b>" + txtName.Text + "<br/>"
+ "<b>Sender Email : </b>" + txtEmail.Text + "<br/>"
+ "<b>Comments : </b>" + txtComments.Text;
mailMessage.IsBodyHtml = true;
SmtpClient smtpClient = new SmtpClient("smtp.gmail.com", 587);
smtpClient.EnableSsl = true;
smtpClient.Credentials = new
System.Net.NetworkCredential("YourGmailID@gmail.com","YourGmailPassowrd");
smtpClient.Send(mailMessage);
Label1.ForeColor = System.Drawing.Color.Blue;
Label1.Text = "Thank you for contacting us";
txtName.Enabled = false;
txtEmail.Enabled = false;
txtComments.Enabled = false;
txtSubject.Enabled = false;
Button1.Enabled = false;
}
}
catch (Exception ex)
{
// Log the exception information to
// database table or event viewer
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Text = "There is an unkwon problem. Please try later";
}
}
}
Once the form is submitted, an email should be sent to the support group. The email should contain all the details that are entered in the contact us form.
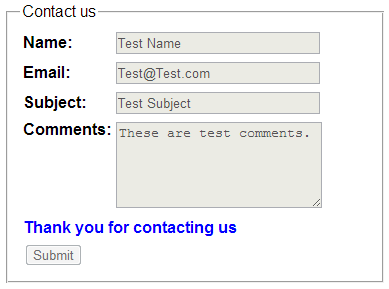

contactus.aspx:
<div style="font-family: Arial">
<fieldset style="width: 350px">
<legend title="Contact us">Contact us</legend>
<table>
<tr>
<td>
<b>Name:</b>
</td>
<td>
<asp:TextBox
ID="txtName"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator1"
runat="server"
ControlToValidate="txtName"
ErrorMessage="Name is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td>
<b>Email:</b>
</td>
<td>
<asp:TextBox
ID="txtEmail"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
Display="Dynamic"
ForeColor="Red"
ID="RequiredFieldValidator2"
runat="server"
ControlToValidate="txtEmail"
ErrorMessage="Email is required"
Text="*">
</asp:RequiredFieldValidator>
<asp:RegularExpressionValidator
Display="Dynamic"
ForeColor="Red"
ID="RegularExpressionValidator1"
runat="server"
ErrorMessage="Invalid Email"
ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"
ControlToValidate="txtEmail"
Text="*">
</asp:RegularExpressionValidator>
</td>
</tr>
<tr>
<td>
<b>Subject:</b>
</td>
<td>
<asp:TextBox
ID="txtSubject"
Width="200px"
runat="server">
</asp:TextBox>
</td>
<td>
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator3"
runat="server"
ControlToValidate="txtSubject"
ErrorMessage="Subject is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td style="vertical-align: top">
<b>Comments:</b>
</td>
<td style="vertical-align: top">
<asp:TextBox
ID="txtComments"
Width="200px"
TextMode="MultiLine"
Rows="5"
runat="server">
</asp:TextBox>
</td>
<td style="vertical-align: top">
<asp:RequiredFieldValidator
ForeColor="Red"
ID="RequiredFieldValidator4"
runat="server"
ControlToValidate="txtComments"
ErrorMessage="Comments is required"
Text="*">
</asp:RequiredFieldValidator>
</td>
</tr>
<tr>
<td colspan="3">
<asp:ValidationSummary
HeaderText="Please fix the following errors"
ForeColor="Red"
ID="ValidationSummary1"
runat="server" />
</td>
</tr>
<tr>
<td colspan="3">
<asp:Label
ID="lblMessage"
runat="server"
Font-Bold="true">
</asp:Label>
</td>
</tr>
<tr>
<td colspan="3">
<asp:Button
ID="Button1"
runat="server"
Text="Submit"
OnClick="Button1_Click" />
</td>
</tr>
</table>
</fieldset>
</div>
contactus.aspx.cs
public partial class contactus : System.Web.UI.Page
{
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (Page.IsValid)
{
MailMessage mailMessage = new MailMessage();
mailMessage.From = new MailAddress("YourGmailID@gmail.com");
mailMessage.To.Add("AdministratorID@YourCompany.com");
mailMessage.Subject = txtSubject.Text;
mailMessage.Body = "<b>Sender Name : </b>" + txtName.Text + "<br/>"
+ "<b>Sender Email : </b>" + txtEmail.Text + "<br/>"
+ "<b>Comments : </b>" + txtComments.Text;
mailMessage.IsBodyHtml = true;
SmtpClient smtpClient = new SmtpClient("smtp.gmail.com", 587);
smtpClient.EnableSsl = true;
smtpClient.Credentials = new
System.Net.NetworkCredential("YourGmailID@gmail.com","YourGmailPassowrd");
smtpClient.Send(mailMessage);
Label1.ForeColor = System.Drawing.Color.Blue;
Label1.Text = "Thank you for contacting us";
txtName.Enabled = false;
txtEmail.Enabled = false;
txtComments.Enabled = false;
txtSubject.Enabled = false;
Button1.Enabled = false;
}
}
catch (Exception ex)
{
// Log the exception information to
// database table or event viewer
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Text = "There is an unkwon problem. Please try later";
}
}
}
Once the form is submitted, an email should be sent to the support group. The email should contain all the details that are entered in the contact us form.
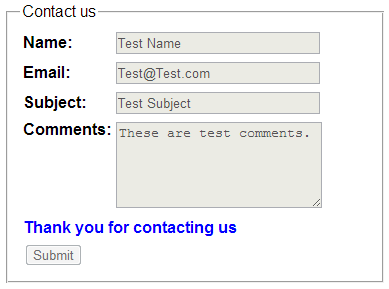
No comments:
Post a Comment